Getting started with Git and BitBucket on Windows
This post describes setting up Git on Windows and using a private remote Git repository hosted on BitBucket.org. Here it goes:
1) Start by downloading Git fromthe msysgit site. At the time of writing the latest installer was Git-1.8.1.2-preview20130201.exe. For some unknown reason the installers are labeled “preview” which normally suggests unfinished product, but the versions are actually fully functional.
After downloading, run the installer and follow the steps. You may use the default options on each screen, but since we are going to try TortoiseGit later, on the first screen select “Simple context menu (Registry based)” and check only “Git Bash Here”. That will keep to your Windows Explorer context menu less cluttered.
2) Install TortoiseGit. At the time of this writing the latest version was 1.8.1. There is nothing special about installing TortoiseGit.
To verify the TortoiseGit installer integrated with your msysgit installation: right click anywhere in Windows Explorer and select TorstoiseGit > Settings from the context menu, then make sure you have the correct paths for Git.exe under the General section and the ssh client under the Network section.
3) Install the Credential Caching Git extension. We will be using Git via https://
instead of ssh
and that extension caches the username and password, so we do not have to type them all the time. Download git-credential-winstore and GitPad for Windows 8 (.NET 4.0 required), unzip,copy git-credential-winstore.exe to the Git bin directory and run it. By default Git is installed in C:\Program Files (x86)\Git\bin
on 64 Bit systems. Open your global .gitconfig file. The global .gitconfig file is in your home directory which on Windows Vista and above should be under C:\Users\<yourname>
. Verify the you have this line in .gitconfig*:
4) Optionally you may want to change the default text editor that Git uses, e.g. you might need that for commit messages. The default editor in msysgit is Unix VIM. You can change that to Notepad with GitPad – a small utility found in the credential caching extension zip. Simply copy GitPad.exe inC:\Program Files (x86)\Git\bin and run it.
5) Create an empty Git repository onBitbucket.org – that will be your shared repository. If you are new to BitBucket you can check this shorttutorialon how to create a BitBucket account and a Git repo- the process is rather straightforward. For this demo create a repo and name it SandboxGit. Please note that BitBucket will create a bare repository. A bare repository is one without branches.
6) We are now ready to start playing with the SandboxGit repo. If you are new to Git, it is better to start in Git Bash at the beginning just to get a feeling of Git. Knowing Git command line will help you understand what TortoiseGit or any other GUI tool does later, because in almost all cases, all the GUI tools generate and execute Git commands for you.
Configure default name and email for commits
In Windows Explorer, go to a place where you want to put your working directory, right click and selectGit Bash Herefrom the menu.
In Git Bash prompt enter:
After the changes, C:\Users\<yourname>\.gitconfig
should have the user configuration in it:
[credential]
helper = !~/AppData/Roaming/GitCredStore/git-credential-winstore
[user]
name = user
email = user@domain.com
Also we can get the same result with the git config
command:
git config --global -l \
credential.helper=!~/AppData/Roaming/GitCredStore/git-credential-winstore \
user.name=user \
user.email=user@domain.com
Configure / disable line ending conversion
Without that we will get CR/LF conversion warning on each commit, e.g. something like this will be shown for each new line in your source:
warning: LF will be replaced by CRLF in HelloWorld.cpp.
The file will have its original line endings in your working directory.
Modern development tools support both CR/LF and LF line endings, so we do not need Git to do line ending conversion.
Clone the remote repository
git clone https://user@bitbucket.org/primosoftware/sandboxgit.git ./SandboxGit
Cloning into './SandboxGit'...
warning: You appear to have cloned an empty repository.
The first time you do this Git will ask for a BitBucket.org password. After that it will remember the password, because of the credentials caching extension thatwe installed in step 3.
Check the remote configuration
``` bash cd ./SandboxGit git remote show origin
``` bash
git remote show origin
* remote origin
Fetch URL: https://user@bitbucket.org/primosoftware/sandboxgit.git
Push URL: https://user@bitbucket.org/primosoftware/sandboxgit.git
HEAD branch: (unknown)
Local branch configured for 'git pull':
master merges with remote master
Basically we have:
- An
origin
alias that points to a remote repo at https://user@nullbitbucket.org/primosoftware/sandboxgit.git - A local branch
master
that will merge with the remote branchmaster
. However, the remotemaster
branch does not exist yet. It will be created when we do our first commit and push. - A remote HEAD branch that is unknown.
Think of HEAD as a symlink/shortcut to a branch. The HEADis unknown because there are no branches in the remote repository yet. Normally HEAD points to themasterbranch.
Add a file and commit
In the SandboxGit folder create a fileHelloWorld.cppwith some code in it. For example:
#include <iostream>
using namespace std;
void main()
{
cout << "Hello World!" << endl;
cout << "Welcome to Git" << endl;
}
In Git Bash do git add HelloWorld.cpp
:
git commit
– enter “Initial commit.” when Git asks for a commit message.
git commit .
[master (root-commit) 8ed71ed] Initial commit.
1 file changed, 7 insertions(+)
create mode 100644 HelloWorld.cpp
The commit is local. Up to this point we have done local changes only. The remote repository on BitBucket.org is still untouched.
In order for others to see the changes we have to push the changes to the remote location. That is done with thegit push command.
Configure default “push” style
Git pushcan work in one of two ways:
- matching: pushes all local branches to remote branches with the same name. This may lead to unwanted remote branches created by accident.
- simple: pushes only the current local branch to its remote brother which is usually a remote branch with the same name. This is a more intuitive behavior.
We will use the simple push:
Push to the remote repository
git push
Counting objects: 3, done.
Delta compression using up to 8 threads.
Compressing objects: 100% (2/2), done.
Writing objects: 100% (3/3), 322 bytes, done.
Total 3 (delta 0), reused 0 (delta 0)
remote: bb/acl: user is allowed. accepted payload.
To https://user@bitbucket.org/primosoftware/sandboxgit.git
* [new branch] master -> master
Verify that now we have a remote branch called master:
``` bash git remote show origin * remote origin Fetch URL: https://user@bitbucket.org/primosoftware/sandboxgit.git Push URL: https://user@bitbucket.org/primosoftware/sandboxgit.git HEAD branch: master Remote branch: master tracked Local branch configured for 'git pull': master merges with remote master Local ref configured for 'git push': master pushes to master (up to date)
We are done.
#### Configure default name and email for commits
Right click anywhere in Windows Explorer and select **TorstoiseGit > Settings** from the context menu, then enter your name and email on under the **Git** section.
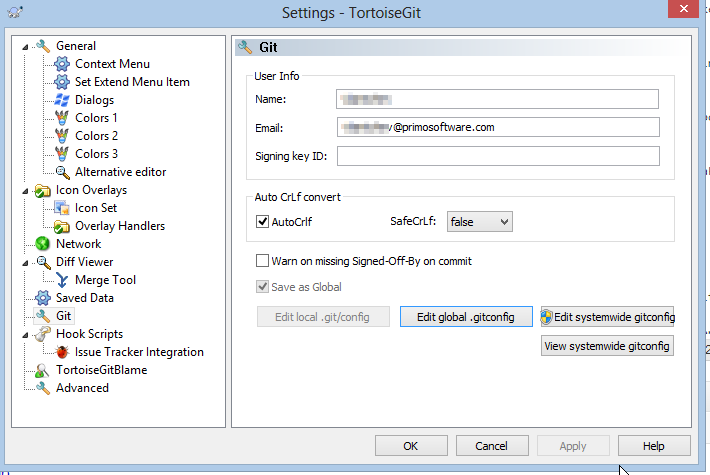
Click *Edit global .gitconfig*. Verify that the *.gitconfig* file now contains the user configuration:
``` ini
[credential]
helper = !~/AppData/Roaming/GitCredStore/git-credential-winstore
[user]
name = user
email = user@domain.com
Configure / disable line ending conversion
Again in the TorstoiseGit > Settings > Git section, in the "Auto CrLf convert" group, uncheck the AutoCrlf box.
Without that we will get CR/LF conversion warning on each commit, e.g. something like this will be shown for each new line in your source code:
warning: LF will be replaced by CRLF in HelloWorld.cpp.
The file will have its original line endings in your working directory.
Modern development tools support both CR/LF and LF line endings, so we do not need Git to do line ending conversion.
Clone the remote repository
In Windows Explorer, go to a place where you want to put your working directory, right click and select Git Clone from the menu.
Check the remote configuration
Right click on the SandboxGit
folder in Windows Explorer and select TorstoiseGit > Settings from the context menu, then go to the Git > Remote section. Verify the remote url to which the origin alias points.
Add a file and commit
In the SandboxGit folder create a fileHelloWorld.cppwith some code in it. For example:
#include <iostream>
using namespace std;
void main()
{
cout << "Hello World!" << endl;
cout << "Welcome to Git" << endl;
}
Right click on the HelloWorld.cpp
file and select TortoiseGit > Add. Check the HelloWorld.cpp
file and click OK.
Right click on the HelloWorld.cpp
file again and select Git Commit -> Master. Enter “Initial commit.” for a message. Check the HelloWorld.cpp
file and click OK.
The commit is local. Up to this point we have done local changes only. The remote repository on BitBucket.org is still untouched.
Push to the remote repository
Right click on theSandboxGitfolder in Windows Explorer and select TorstoiseGit > Push from the context menu. Click OK.
We are done.